You may need to let your business users edit the physical files that your media items point to. Since reuploading a file will cause creation of another media item and you will have to reconnect the content item with media item, this could be too complex for business users.
This then the following approach can be used.
First of all, a special button should be added to the media tab. In order to do this, go to the core database -> and create a button under /sitecore/system/Ribbons/Contextual Ribbons/Media/Media/Media. The easiest way will be to duplicate the existing Download button and change the name to something more appropriate, e.g. "Edit File". Set the following properties:
- Header = Edit File
- Icon = Applications/16x16/contract.png
- Click = media:edit
- Tooltip = Edit the file.
The Click field is the most important. This value will connect the button with the execution logic.
Next step will be to define the "media:edit" command. Open the \app_config\Commands.config file and add something like:
<command name="media:edit" type="WebApp.Shell.Framework.Commands.Media.Edit,WebApp" />
This will tell Sitecore to look up the "WebApp.Shell.Framework.Commands.Media.Edit" class in the WebApp assembly from the bin folder.
So the only thing that is left to do is to compile the code that will execute an application to edit the file.
The code is represented below:
using Sitecore;
using Sitecore.Data;
using Sitecore.Data.Items;
using Sitecore.Shell.Framework;
using Sitecore.IO;
using Sitecore.Text;
using Sitecore.Shell.Framework.Commands;
namespace WebApp.Shell.Framework.Commands.Media
{
public class Edit : Command
{
public override void Execute(CommandContext context)
{
foreach (Item item in context.Items)
{
string filename = item["file path"];
if (FileUtil.FileExists(filename))
{
UrlString url = new UrlString();
url.Append("fi", filename);
if (filename.EndsWith(".xml", StringComparison.OrdinalIgnoreCase))
{
Windows.RunApplication("Layouts/IDE", url.ToString());
return;
}
}
}
}
}
}
This is basically a class that inherits from the Command base class and contains one single method "Execute" that is fired whenever the button is clicked.
In the logic of this method we simply get the currently selected media item, get the physical path and check if this is an XML file. If it is, we execute the Developer Center application that allows us to edit and save the file. You can modify the logic to fit your needs.
This is how it looks like:
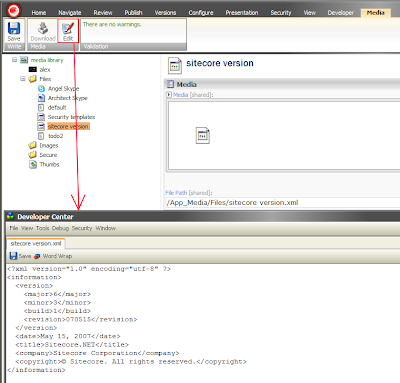
Note that this scenario deals with file system media storage. In case of database storage you will be able to download the media file from the blob field and reattach it to the same media item.