<?xml version="1.0" encoding="utf-8" ?>
<control xmlns:def="Definition" xmlns="http://schemas.sitecore.net/Visual-Studio-Intellisense">
<PreviewCurrentWorkflowPortletdef:inherits="Custom.Portlets.PreviewCurrentWorkflowPortletXmlControl,CurrentWorkflowPortlet">
<Border def:ID="Portlet"><DefaultPortletWindow def:ID="Window" Header="Workflow Details" Icon="Network/16x16/inbox.png">
<Border def:ID="Body"/><Literal def:ID="Workflow" Text="Workflow: " />
<br /><Literal def:ID="WorkflowState" Text="Workflow State" />
</DefaultPortletWindow></Border>
</PreviewCurrentWorkflowPortlet></control>
6. Create a class that will stand for this XML control. Compile it and place into the bin folder. using System; using Sitecore; using Sitecore.Data.Items; using Sitecore.Web.UI.XmlControls; using Sitecore.Web.UI.HtmlControls; namespace Custom.Portlets { public class PreviewCurrentWorkflowPortletXmlControl : XmlControl { protected Border Body; protected Border Portlet; protected XmlControl Window; protected Literal WorkflowState; protected Literal Workflow; protected override void OnLoad(EventArgs e) { base.OnLoad(e); if (!Sitecore.Context.ClientPage.IsEvent) { this.Window.ID = this.ID + "_window"; this.Portlet.Attributes["id"] = this.ID; Item item = UIUtil.GetItemFromQueryString(Sitecore.Context.ContentDatabase); string workflowID = item.Fields["__Workflow"].Value; string stateName = "none"; string workflowName = "none"; if(workflowID != "") { stateName = GetState(item, Sitecore.Context.Database, workflowID).DisplayName; workflowName = Sitecore.Context.Database.Items[new Sitecore.Data.ID(workflowID)].Name; } this.WorkflowState.Text += stateName; this.Workflow.Text += workflowName; } } private Sitecore.Workflows.WorkflowState GetState(Sitecore.Data.Items.Item item, Sitecore.Data.Database database, string workflowID) { // getting the workflow provider for the master database Sitecore.Workflows.IWorkflowProvider provider = database.WorkflowProvider; // getting the Simple workflow through the IWorkflow interface Sitecore.Workflows.IWorkflow iWorkflow = provider.GetWorkflow(workflowID); return iWorkflow.GetState(item); } } } 7. Add the reference to this dll into the web.config (UI » References section):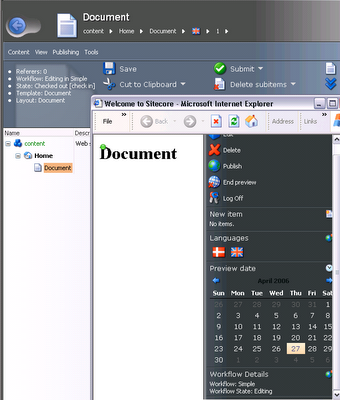