This is some instructions on how to add an Export button to the Broken Links application. Upon clicking, you can export the results of the broken link search to any location, e.g. Excel worksheet, XML file, etc. The sample code below outputs the results to the log file.
1. Add a command under /content/applications/tools/broken links/commands:
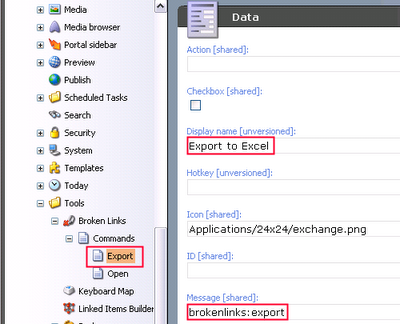
2. Fill the fields as it is shown above.
3. Find the XML control that stands for the Broken Link dialog. It can be found here:
\sitecore\shell\Applications\Tools\Broken links\Broken links.xml
4. Substitute the code beside definition with your custom class as it is shown below:
<CodeBeside Type="Custom.CustomBrokenLinksForm,BrokenLinksForm"/>
where Custom.CustomBrokenLinksForm is the full class name with namespace,
BrokenLinksForm is the name of the assembly.
5. Create and compile the following sample code into the bin directory.
This class outputs the broken link results into the log file. You can implement your own logic here to export it to any file.
using System;
using Sitecore;
using Sitecore.Jobs;
using Sitecore.Links;
using Sitecore.Web.UI.Sheer;
using Sitecore.Resources;
using Sitecore.Diagnostics;
namespace Custom
{
public class CustomBrokenLinksForm : Sitecore.Shell.Applications.Tools.BrokenLinks.BrokenLinksForm
{
[HandleMessage("brokenlinks:export")]
protected void Export(Message message)
{
Context.ClientPage.ClientResponse.Timer("StartExport", 10);
}
public void StartExport()
{
Context.ClientPage.ServerProperties["handle"] = LinkDatabaseProxy.GetBrokenLinks(Context.ContentDatabase).ToString();
CheckExportStatus();
}
protected void CheckExportStatus()
{
Handle handle = Handle.Parse(Context.ClientPage.ServerProperties["handle"] as string);
JobStatus status = LinkDatabaseProxy.GetStatus(handle);
if (status.Failed)
{
Sitecore.Context.ClientPage.ClientResponse.ShowError("An error occured.", StringUtil.StringCollectionToString(status.Messages));
}
else if (status.State == JobState.Finished)
{
ExportReport(status);
}
else
{
Context.ClientPage.ClientResponse.SetInnerHtml("Report", "
" + Images.GetImage("Applications/48x48/exchange.png", 0x30, 0x30) + "
Processed: " + status.Processed.ToString() + " |
");
Context.ClientPage.ClientResponse.Timer("CheckExportStatus", 500);
}
}
protected void ExportReport(JobStatus status)
{
ItemLink[] linkArray = status.Result as ItemLink[];
Log.Info("Exporting the broken link results into Excel worksheet...", this);
if(linkArray.Length == 0)
{
Log.Info("There were no broken links found. ", this);
}
else
{
foreach(ItemLink itemLink in linkArray)
{
Log.Info(String.Format("Broken link found. SourceDatabaseName: {0}, SourceFieldID: {1}, SourceItemID: {2}, TargetDatabaseName: {3}, TargetItemID: {4}, TargetPath: {5}.",
itemLink.SourceDatabaseName,
itemLink.SourceFieldID,
itemLink.SourceItemID,
itemLink.TargetDatabaseName,
itemLink.TargetItemID,
itemLink.TargetPath), this);
}
}
Context.ClientPage.ClientResponse.SetInnerHtml("Report", "The export process is finished.");
}
}
}
0 comments:
Post a Comment